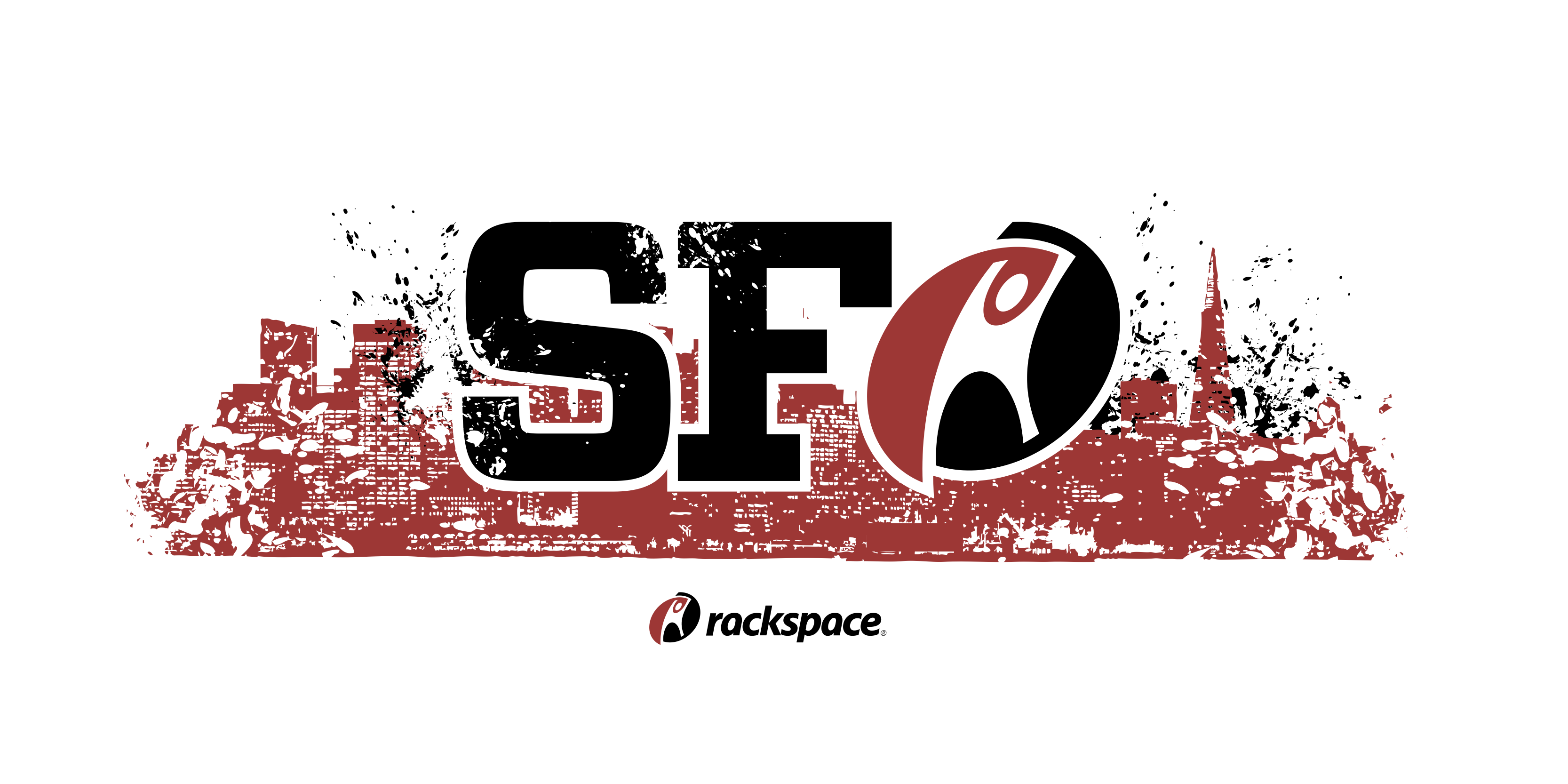
Awareness of the system
The goal:
push to monitoring server
examples of internal monitors
{ target = "endpoint", source = "agentA", id = 0, params = { agent_id = "agentA", process_version = "f451d7097edb197a9e08fa05cf5b0556ed15d7c7", token = "0000000000000000000000000000000000000000000000000000000000000000.7777", bundle_version = "0.1-75-gf451d70" }, v = "1", method = "handshake.hello" }
{ target = "agentA", source = "endpoint", id = 0, result = { heartbeat_interval = 1000 }, v = "1" }
{ "v": "1", "id": 2, "source": "endpoint", "target": "agentA", "result": { "checks": { "id": "ch1234", "type": "agent.cpu", "details": { "foo": "foo" }, "period": 30, "timeout": 30, "disabled": false } }, "error": null }
Luvit is a platform for building your app
local http = require("http") http.createServer(function (req, res) local body = "Hello world\n" res:writeHead(200, { ["Content-Type"] = "text/plain", ["Content-Length"] = #body }) res:finish(body) end):listen(8080) print("Server listening at http://localhost:8080/")
GroundControl = {} function GroundControl.new() obj = {} obj.heard_major_tom = false setmetatable(obj, { __index = GroundControl }) return obj end function GroundControl:heard() print(self.heard_major_tom) end a = GroundControl.new() a:heard() a.heard() -- this will error
while (1) { nfds = poll(fds, next_timer()); if (nfds == 0) timer_callback(); for(n = 0; n < nfds; ++n) { if (fds[n] == READY) callbacks[n](); } }
http://github.com/racker/virgo